Designing User-Defined Methods in Java: Key Criteria
- Alex Ricciardi
- Oct 23, 2024
- 3 min read
This post explores the criteria for designing user-defined methods in Java, focusing on functionality, reusability, maintainability, and clarity. It provides an example of a discount calculator method, illustrating how thoughtful design can address specific business needs while promoting efficient and maintainable code.
Alexander S. Ricciardi
May 20, 2024
Java, like other Object-Oriented Programming (OOP) languages, allows user-defined methods (functions) which provide developers with the flexibility to create methods that address the specific needs of an application. When designing a method, it is crucial to carefully consider the rationale, limitations, and criteria specific to each application while ensuring functionality, reusability, maintainability, and clarity.
An example of a user-defined function/method that can be used to elaborate on the criteria utilized to develop the method and its rationale could be a method that calculates the discount for a customer based on loyalty points, seasonal promotions, and purchase history. Here are four criteria and rationales that I think should be used to develop the method:
Define the task – Method functionality:
If the task involves unique business logic or specific requirements that cannot be addressed by Java predefined methods, a user-defined method is necessary. In the case of the method described above, its task is to calculate the discount for a customer based on loyalty points, seasonal promotions, and purchase history.
Rationale: Unique business logic and specific requirements often are required to develop appropriate solutions to a need or a problem that predefined methods cannot offer. User-defined function/method ensures that the solution meets the business needs.
Task reusability – The method will be reused throughout the codebase:
The task functionality will be reused in multiple parts of the application. The method can be called across different modules without duplicating code.
Rationale: Reusability promotes modularity and reduces code duplication, ensuring consistent implementation of the discount calculation logic throughout the application.
Future modifications – Method maintainability:
Over time, the task’s functionality may need to change; for example, the number of loyalty points required to affect the discount calculation may vary in the future. The methods improve maintainability by encapsulating specific logic in one block of code. This makes it easier to update/change and debug the code.
Rationale: Encapsulation of code logic and functionality within a method makes maintainability possible and easier. This makes future updates or modifications simpler and reduces the risk of introducing errors.
Task describing – Naming, parameters, Javadocs, and comments:
Documentation is needed to describe the task and define the task parameters. Naming a method appropriately, choosing/declaring clear parameters, and using Javadocs and comments are crucial for code readability/understandability and defining parameters.
Rationale: Well-documented code with clear naming conventions and parameter declaration and improves code functionality, readability, and helps other developers understand the purpose and functionality of the method.
Code Example:
/**
* The DiscountCalculator class provides functionality to calculate discounts
* for customers based on loyalty points, seasonal promotions, and purchase history.
*/
public class DiscountCalculator {
/**
* Calculates the discount for a customer based on loyalty points,
* seasonal promotions, and purchase history.
*
* @param loyaltyPoints (int) The number of loyalty points.
* @param seasonalPromo (Double) The seasonal promotion discount percentage.
* @param purchaseHistory (double) The customer's total purchase history amount.
* @return (double) The calculated discount amount.
*/
public double calculateDiscount(int loyaltyPoints, double seasonalPromo, double
purchaseHistory) {
double baseDiscount = 0.0;
// Add loyalty points discount
baseDiscount += loyaltyPoints * 0.01;
// Apply seasonal promotion
baseDiscount += seasonalPromo;
// Additional discount based on purchase history
if (purchaseHistory > 1000) {
baseDiscount += 5.0; // Additional 5% discount for high spenders
}
return baseDiscount;
}
}
It is also important to understand when designing the method vocabulary specific to each programming language, below is Java method vocabulary:
Figure 1
Java Method Vocabulary
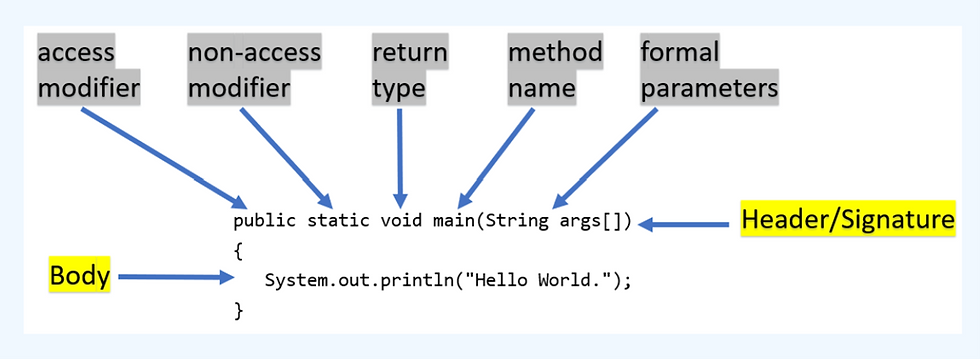
Note: From "Introduction to Programming with Java: 5.1.1 Writing Static Methods" by Ericson et al., 2015
To summarize, user-defined methods, such as the discount calculator, demonstrate the importance of balancing functionality, modularity, maintainability, and documentation in software design while carefully considering the rationale, limitations, and criteria specific to each application. By defining specific tasks, promoting reusability, planning for future modifications, and ensuring clear naming and documentation, developers can create methods that are not only effective but also adaptable to changing requirements.
References:
Ericson, B. (2015). Introduction to programming with Java: 5.1.1 Writing static methods [Image]. Runestone Academy. https://runestone.academy/ns/books/published/csjava/Unit5-Writing-Methods/topic-5-1-writing-methods.html
Comments