This article explains how to use Python's data types effectively to create scalable and maintainable applications.
Alexander S. Ricciardi
February 21th, 2024
Python offers a rich variety of data types that are fundamental to writing effective and efficient code. Understanding these data types is crucial for any developer, as it allows for proper data storage, manipulation, and retrieval. In this guide, we’ll explore common Python data types, their applications, and strategies for determining which data types to use in different scenarios.
A quick explanation of Python data types.
First, Python offers a vast array of data types. The Python documentation provides detailed descriptions of each data type, and you can find the list at the following link: Data Types. “Python also provides some built-in data types, in particular, dict, list, set, and frozenset, tuple. The str class is used to hold Unicode strings, and the bytes and bytearray classes are used to hold binary data” (Python Software Foundation (a), n.d., Data Type). Built-in data types in Python are fundamental data structures that come standard with Python; you don't need to import any external library to use them.
The table below shows Python's common data types.
Table-1
Common Data Types
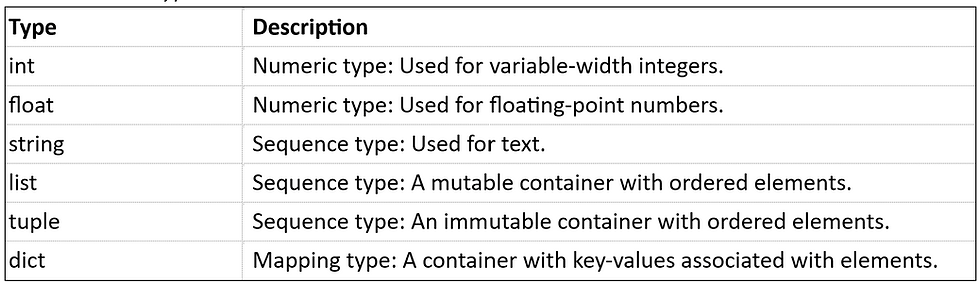
Note: from Programming in Python 3, by Bailey, 2016.
Strategy for Determining Data Types
To determine the data types needed for an application, it is crucial to analyze the data that needs to be collected and understand the application's functionality requirements. In general, this equates to these four key steps:
Identifying the Data: identifying what types of data the application will collect and handle, such as textual information and numerical data.
Understanding Data Operations: which operations will be performed on the data, such as sorting, searching, or complex manipulations, to ensure the chosen data types can support these functionalities.
Structuring Data Relations: how different pieces of data relate to each other and deciding on the appropriate structures (e.g., nested dictionaries or lists) to represent these relationships efficiently.
Planning for Scalability and Maintenance: future expansions or modifications to the application and selecting data types and structures that allow for modification, updates, and scalability.
For this specific application, this translates to the following steps:
Note that the information provided does not explicitly state whether the data needs to be manipulated (sorted or modified). However, for the application to be useful and functional, the data needs to be manipulated to some extent.
Based on the information provided, the application functionality requirements are as follows:
Storing Personal Information: storing basic personal information for each family member, such as names and birth dates.
Address Management: manage and store current and possibly multiple addresses for each family member.
Relationship Tracking: tracking and representing the relationships between different family members (e.g., parent-child, spouses, siblings).
Data Manipulation: functionalities for editing, sorting, and updating the stored information, including personal details, addresses, and family relationships.
Based on the information provided, the data that needs to be collected is as follows:
Names: this includes names and family members' names are text data
Birth dates: birth dates can be text data, numbers data, or a mix of both.
Address: addresses can be complex, potentially requiring storage of multiple addresses per family member with components like street, city, state, and zip code. It is a mix of numbers and text data.
Relationship: relationships between family members (e.g., parent-child, spouses, siblings) is text data.
Four data elements and the corresponding data types
Taking into account the application functionality requirements and data information the following are the four data elements and the corresponding data types.
Names: the string data type str. This allows us to easily store and manipulate individual names. I will use a tuple to separate the first name and last name, name = (‘first_name’, ‘last_name’). Tuples are great in this case because they are immutable, meaning once a tuple is created, it cannot be altered ensuring that the integrity of first and last names is preserved. Additionally, they are indexed meaning that they can be searched by index. For example, a list name tuple can be searched by last or first name. Furthermore, a tuple takes less space in memory than a dictionary or a list.
Birth Dates: they could technically be stored as strings, integers, lists, or dictionaries, however utilizing the datetime.date object from Python's datetime module has significant advantages such as easy date manipulations and functionality. For example, calculating ages, or sorting members by their birth dates. In most cases storing birth dates, requires converting input strings into datetime.date objects. Note that datetime is a class. Additionally, in Python data types (floats, str, int, list, tuple, set, ...) are instances of the Python `object`. In other words, they are objects.
A datetime.date object utilizes the following data type :
Year: An integer representing the year, e.g., 2024.
Month: An integer representing the month, from 1 (January) to 12 (December).
Day: An integer representing the day of the month, from 1 to 31, depending on the month and year.
For example: Note: the method date.fromisoformat() coverts strings into datetime.date() object with integer arguments.
from datetime import date
>>> date.fromisoformat('2019-12-04')
datetime.date(2019, 12, 4)
>>> date.fromisoformat('20191204')
datetime.date(2019, 12, 4)
>>> date.fromisoformat('2021-W01-1')
datetime.date(2021, 1, 4)
(Python Software Foundation (b), n.d., datetime - Basic date and time types )
Address: addresses have multiple components such as street, city, state, and zip code. I would use a dictionary data type dict. The dictionary key-value pair items structure is great for storing, modifying, and accessing the various parts of an address.
Relationship: relationships between family members, such as parent-child, spouses, and siblings. I would use a dictionary data type dict with embedded List and tuple data types. In this structure, the keys represent the types of relationships, and the values are lists of names or identifiers referencing other family members. This would allow for easy storing, modifying, and accessing relationships data.
Below is an example of what the Python code could be like:
from datetime import date
user_123 = {
"name": ("John", "Doe"), # Using tuple for the name
"birth_date": date(1974, 6, 5), # Using datetime for birth dates
"address": { # Using a dictionary for the address
"street": "123 My Street",
"city": "Mytown",
"state": "Mystate",
"zip_code": "12345"
},
"relationships": { # Using a dictionary with embedded lists and tuples
"spouse": ("Jane", "Doe"),
"children": [("George", "Doe"), ("Laura", "Doe")],
"parents": [("Paul", "Doe"), ("Lucy", "Doe")],
}
}
To create well-structured and maintainable applications in Python, it is essential to choose the right data types. To ensure your code is both efficient and scalable, it’s crucial to understand the differences between Python’s built-in data types—such as strings, tuples, dictionaries, and datetime objects—and to implement them effectively
References:
Bailey, M. (2016, August). Chapter 3: Types, Programming in Python 3. Zyante Inc.
Python Software Foundation (a). (n.d.). Data Type. Python.
Python Software Foundation (b). (n.d.). datetime - Basic date and time types Python. python.org. https://docs.python.org/3/library/datetime.html
Comments